Welcome, Guest |
You have to register before you can post on our site.
|
Online Users |
There are currently 1341 online users. » 0 Member(s) | 1338 Guest(s) Yandex, Bing, Applebot
|
Latest Threads |
SELECT statement with MS ...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:35 PM
» Replies: 0
» Views: 1,478
|
SELECT statement with the...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:31 PM
» Replies: 0
» Views: 853
|
Creating hyperlinks in HT...
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 01:23 PM
» Replies: 0
» Views: 1,214
|
What's new in HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:48 PM
» Replies: 0
» Views: 907
|
What is HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:43 PM
» Replies: 0
» Views: 822
|
Neck isometric exercises
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:44 AM
» Replies: 0
» Views: 1,166
|
Shoulder shrug
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 748
|
Neck retraction
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 731
|
Neck flexion and extensio...
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 824
|
Neck rotation
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 793
|
|
|
JavaScript exercises and solutions - Swap two numbers |
Posted by: Qomplainerz - 04-04-2023, 07:54 AM - Forum: JavaScript Tutorials
- No Replies
|
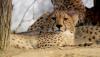 |
The HTML part between the <body></body> tags looks like this:
Code: Enter the first number here:
<br>
<input type="Text" id="Num1">
<br>
Enter the second number here:
<br>
<input type="Text" id="Num2">
<br>
<input type="button" id="submit" value="submit" onclick="SwapNumbers()"></input>
<p id="Output"></p>
<input type="button" id="reset" value="Reset" onclick="Reset()"></input>
The JavaScript part looks like this:
Code: function SwapNumbers()
{
Num1 = document.getElementById("Num1").value;
Num2 = document.getElementById("Num2").value;
Num3 = Num1;
Num4 = Num2;
Output = "You have entered " + Num4 + " and " + Num3;
document.getElementById("Output").innerHTML = Output;
}
function Reset()
{
document.getElementById("Num1").value = "";
document.getElementById("Num2").value = "";
document.getElementById("Output").innerHTML = "";
}
Note: The reset button and the reset function are optional in case you want to delete what you were looking for in order to look for something else.
|
|
|
JavaScript exercises and solutions - Iterating through an array with keys and values |
Posted by: Qomplainerz - 04-04-2023, 07:52 AM - Forum: JavaScript Tutorials
- No Replies
|
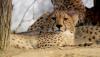 |
Code: const Games =
[
"Assetto Corsa",
"Assetto Corsa Competizione",
"rFactor",
"rFactor2",
"Kart Racing Pro",
"KartKraft",
"vRally",
"WRC 10",
"F1 2023",
"F1 2022",
"F1 2021",
"F1 2020",
"F1 2019",
"F1 2018",
"F1 2017",
"F1 2016",
"F1 2015",
"F1 2014",
"F1 2013",
"F1 2012",
];
for(i = 0; i < Games.length; i++)
{
document.write(i + " ");
document.write(Games[i] + "<br>");
}
|
|
|
JavaScript - Switch Break Statements |
Posted by: Qomplainerz - 04-04-2023, 07:47 AM - Forum: JavaScript Tutorials
- No Replies
|
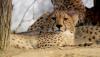 |
The HTML part between the <body></body> tags looks like this:
Code: What's your favorite fruit?
<br>
<input type="text" id="fruit"></input>
<br>
<input type="button" id="submit" onclick="submit()" value="submit"></button>
<br>
<p id="output"></p>
The JavaScript part looks like this:
Code: function submit()
{
fruitType = document.getElementById("fruit").value;
switch(fruitType)
{
case "Oranges":
document.getElementById("output").innerHTML = "Oranges are $0.59 a pound.";
break;
case "Apples":
document.getElementById("output").innerHTML = "Apples are $0.32 a pound.";
break;
case "Bananas":
document.getElementById("output").innerHTML = "Bananas are $0.48 a pound.";
break;
case "Cherries":
document.getElementById("output").innerHTML = "Cherries are $3.00 a pound.";
break;
case "Mangoes":
document.getElementById("output").innerHTML = "Mangoes are $0.56 a pound.";
break;
case "Papayas":
document.getElementById("output").innerHTML = "Papayas are $2.79 a pound.";
break;
default:
document.getElementById("output").innerHTML = `Sorry, we ran out of ${fruitType}. <br> Would you like to get anything else?`;
}
}
|
|
|
Binary to Decimal in JavaScript |
Posted by: Qomplainerz - 04-04-2023, 07:46 AM - Forum: JavaScript Tutorials
- No Replies
|
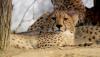 |
Code: Num001 = 0B00000000;
Num002 = 0B00000001;
Num003 = 0B00000010;
Num004 = 0B00000011;
document.write(Num001);
document.write("<br>");
document.write(Num002);
document.write("<br>");
document.write(Num003);
document.write("<br>");
document.write(Num004);
|
|
|
|