Welcome, Guest |
You have to register before you can post on our site.
|
Online Users |
There are currently 1755 online users. » 0 Member(s) | 1753 Guest(s) Bing, Yandex
|
Latest Threads |
SELECT statement with MS ...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:35 PM
» Replies: 0
» Views: 1,076
|
SELECT statement with the...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:31 PM
» Replies: 0
» Views: 528
|
Creating hyperlinks in HT...
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 01:23 PM
» Replies: 0
» Views: 823
|
What's new in HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:48 PM
» Replies: 0
» Views: 547
|
What is HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:43 PM
» Replies: 0
» Views: 514
|
Neck isometric exercises
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:44 AM
» Replies: 0
» Views: 808
|
Shoulder shrug
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 467
|
Neck retraction
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 433
|
Neck flexion and extensio...
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 501
|
Neck rotation
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 473
|
|
|
Basic arithmetic operations with the double datatype in C |
Posted by: Qomplainerz - 07-26-2023, 06:01 PM - Forum: C 18 Tutorials
- No Replies
|
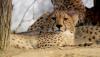 |
Example:
double x = 5.5;
double y = 2.5;
double sum = x + y;
double difference = x - y;
double product = x * y;
double quotient = x / y;
Explanation:
In this example, we declare several double variables (x, y, sum, difference, product, and quotient).
We perform basic arithmetic operations using these variables.
sum stores the result of adding x and y.
difference stores the result of subtracting y from x.
product stores the result of multiplying x and y.
quotient stores the result of dividing x by y.
|
|
|
Double datatype in C |
Posted by: Qomplainerz - 07-26-2023, 06:00 PM - Forum: C 18 Tutorials
- No Replies
|
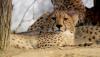 |
Description:
The double data type in C is used to store double-precision floating-point numbers. It typically occupies 8 bytes of memory and offers higher precision compared to the float data type. Double-precision floating-point numbers can represent a broader range of real numbers with greater accuracy.
Example:
double pi = 3.14159265359;
Explanation:
In this example, we declare a variable pi of type double.
We initialize it with the value 3.14159265359.
The double data type provides higher precision than float and can represent real numbers with a range of approximately ±1.8E+308 and typically has 15 to 17 significant decimal digits of precision.
|
|
|
Comparisons with short int in C |
Posted by: Qomplainerz - 07-26-2023, 03:51 PM - Forum: C 18 Tutorials
- No Replies
|
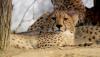 |
Description:
Remember that the short int data type is useful when you need to save memory and the range of values is within its limitations. If you need a broader range or more precision, you may want to use a regular int or other data types like long int or long long int.
Example:
#include <stdio.h>
int main() {
short int a = 10;
short int b = 20;
if (a == b) {
printf("a and b are equal.\n");
} else if (a < b) {
printf("a is less than b.\n");
} else {
printf("a is greater than b.\n");
}
return 0;
}
Explanation:
In this example, we compare two short int values a and b. The program checks whether a is equal to b, less than b, or greater than b, and prints the corresponding message.
|
|
|
Type casting with short int in C |
Posted by: Qomplainerz - 07-26-2023, 03:50 PM - Forum: C 18 Tutorials
- No Replies
|
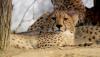 |
Example:
#include <stdio.h>
int main() {
int num1 = 200;
int num2 = 100;
// Type cast integers to short int before addition
short int result = (short int)(num1 + num2);
printf("Result: %d\n", result);
return 0;
}
Explanation:
In this example, we have two integers num1 and num2. Before performing the addition, we type-cast the result of num1 + num2 to a short int using (short int) to fit the result within the range of a short int.
|
|
|
User input and short int conversion in C |
Posted by: Qomplainerz - 07-26-2023, 03:49 PM - Forum: C 18 Tutorials
- No Replies
|
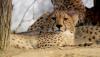 |
Example:
#include <stdio.h>
int main() {
short int userInput;
// Prompt the user to enter a short int value
printf("Enter a short int value: ");
scanf("%hd", &userInput);
// Perform a simple calculation and display the result
short int result = userInput * 2;
printf("Result: %d\n", result);
return 0;
}
Explanation:
In this example, the user is prompted to enter a short int value. The scanf function is used to read the user input as a short int, and it is stored in the userInput variable. Then, we perform a simple calculation (multiplication by 2) using the short int value and display the result using printf.
|
|
|
Overflow and underflow with short int in C |
Posted by: Qomplainerz - 07-26-2023, 03:48 PM - Forum: C 18 Tutorials
- No Replies
|
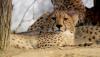 |
Example:
#include <stdio.h>
int main() {
short int max = 32767;
short int min = -32768;
// Overflow example
short int overflowed = max + 1;
printf("Overflowed value: %d\n", overflowed);
// Underflow example
short int underflowed = min - 1;
printf("Underflowed value: %d\n", underflowed);
return 0;
}
Explanation:
In this example, we demonstrate what happens when a short int variable encounters overflow and underflow. The max value for a short int is 32767, and adding 1 to it causes an overflow, resulting in a value of -32768, which is the min value for a short int. Similarly, subtracting 1 from the min value causes an underflow, resulting in 32767, which is the max value for a short int.
|
|
|
Arithmetic operations with short int in C |
Posted by: Qomplainerz - 07-26-2023, 03:46 PM - Forum: C 18 Tutorials
- No Replies
|
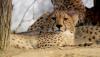 |
Example:
#include <stdio.h>
int main() {
short int a = 1000;
short int b = 200;
// Addition
short int sum = a + b;
printf("Sum: %d\n", sum);
// Subtraction
short int difference = a - b;
printf("Difference: %d\n", difference);
// Multiplication
short int product = a * b;
printf("Product: %d\n", product);
// Division
short int quotient = a / b;
printf("Quotient: %d\n", quotient);
return 0;
}
Explanation:
Explanation: In this example, we perform basic arithmetic operations (addition, subtraction, multiplication, and division) using short int variables. The result of each operation is stored in a short int variable and then printed using the %d format specifier in printf.
|
|
|
Storing short integers in C |
Posted by: Qomplainerz - 07-26-2023, 03:45 PM - Forum: C 18 Tutorials
- No Replies
|
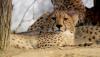 |
Description:
In C, the short int data type is used to represent integer values with a smaller range than regular int. It is a signed integer type that typically occupies 2 bytes in memory, which means it has a smaller range of representable values compared to a regular int.
Example:
#include <stdio.h>
int main() {
// Declare and initialize a short int variable
short int myShortInt = 32767;
// Print the value of the short int variable
printf("Value of myShortInt: %d\n", myShortInt);
return 0;
}
Explanation:
Explanation: In this example, we declare a short int variable called myShortInt and initialize it with the value 32767. The %d format specifier is used in printf to print the value of the short int variable.
|
|
|
Implicit and explicit type conversion in C |
Posted by: Qomplainerz - 07-26-2023, 08:25 AM - Forum: C 18 Tutorials
- No Replies
|
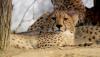 |
Description:
The float data type is useful for representing real numbers with moderate precision. However, it's important to be cautious when comparing float values for equality due to the limited precision. In some cases, you may need to use double data type, which offers higher precision, when working with very small or very large real numbers.
Example:
int intValue = 10;
float floatValue = 2.5;
// Implicit type conversion (promotion)
float result1 = intValue + floatValue; // Result is 12.5
// Explicit type conversion (type casting)
int result2 = (int)(intValue + floatValue); // Result is 12
Explanation:
In this example, we have an int variable intValue with the value 10 and a float variable floatValue with the value 2.5.
In the first expression, intValue is implicitly converted to float during the addition with floatValue. This is called promotion, where the int is promoted to a float to ensure consistent types during the operation.
In the second expression, we use explicit type casting (type conversion) to convert the result of the addition back to an int. The (int) before the expression indicates the type casting operation.
|
|
|
Working with floats from user input in C |
Posted by: Qomplainerz - 07-26-2023, 08:23 AM - Forum: C 18 Tutorials
- No Replies
|
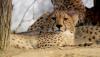 |
Example:
float radius;
printf("Enter the radius of a circle: ");
scanf("%f", &radius);
float area = 3.14159 * radius * radius;
printf("The area of the circle is: %.2f\n", area);
Explanation:
In this example, we declare a float variable radius to store the user's input.
We prompt the user to enter the radius of a circle using printf().
We use the %f format specifier in scanf() to read a floating-point value from the user and store it in the radius variable.
We calculate the area of the circle using the formula area = π * r^2, where π is approximated as 3.14159.
Finally, we print the calculated area using printf(). The .2 in the format specifier %.2f ensures that the area is displayed with two decimal places.
|
|
|
|