Welcome, Guest |
You have to register before you can post on our site.
|
Online Users |
There are currently 1480 online users. » 0 Member(s) | 1477 Guest(s) Yandex, Applebot, Bing
|
Latest Threads |
SELECT statement with MS ...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:35 PM
» Replies: 0
» Views: 1,465
|
SELECT statement with the...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:31 PM
» Replies: 0
» Views: 844
|
Creating hyperlinks in HT...
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 01:23 PM
» Replies: 0
» Views: 1,199
|
What's new in HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:48 PM
» Replies: 0
» Views: 893
|
What is HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:43 PM
» Replies: 0
» Views: 807
|
Neck isometric exercises
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:44 AM
» Replies: 0
» Views: 1,150
|
Shoulder shrug
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 735
|
Neck retraction
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 724
|
Neck flexion and extensio...
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 813
|
Neck rotation
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 787
|
|
|
Infinite recursion in C |
Posted by: Qomplainerz - 07-26-2023, 07:13 AM - Forum: C 18 Tutorials
- No Replies
|
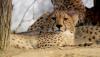 |
Writing recursive functions without a proper base case can lead to infinite recursion.
// Incorrect
void countDown(int n) {
printf("%d ", n);
countDown(n - 1); // Missing base case
}
// Correct
void countDown(int n) {
if (n <= 0) {
return;
}
printf("%d ", n);
countDown(n - 1);
}
|
|
|
Not checking return values in C |
Posted by: Qomplainerz - 07-26-2023, 07:12 AM - Forum: C 18 Tutorials
- No Replies
|
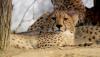 |
Neglecting to check the return values of functions that can fail (e.g., malloc, fopen, etc.) can lead to errors.
// Incorrect
FILE *file = fopen("non_existent_file.txt", "r");
// No check for the success of fopen()
// Correct
FILE *file = fopen("non_existent_file.txt", "r");
if (file == NULL) {
// Handle error (file not opened)
}
|
|
|
Using incorrect format specifiers in C |
Posted by: Qomplainerz - 07-26-2023, 07:11 AM - Forum: C 18 Tutorials
- No Replies
|
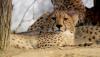 |
Mismatching format specifiers with the data types can lead to undefined behavior or incorrect output.
// Incorrect
int x = 10;
printf("Value of x: %s\n", x); // Incorrect format specifier '%s' for integer
// Correct
int x = 10;
printf("Value of x: %d\n", x); // Output: Value of x: 10
|
|
|
Misusing scanf() in C |
Posted by: Qomplainerz - 07-26-2023, 07:11 AM - Forum: C 18 Tutorials
- No Replies
|
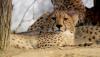 |
Not handling newline characters left in the input buffer after using scanf().
// Incorrect
char name[50];
printf("Enter your name: ");
scanf("%s", name);
// If the user enters "John Doe", the newline character is left in the buffer
// Correct
char name[50];
printf("Enter your name: ");
scanf("%49[^\n]", name);
// The format specifier reads up to 49 characters or until a newline character
|
|
|
Uninitialized pointers in C |
Posted by: Qomplainerz - 07-26-2023, 07:10 AM - Forum: C 18 Tutorials
- No Replies
|
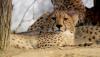 |
Using pointers without initializing them or dereferencing NULL pointers can lead to crashes.
// Incorrect
int *ptr; // Uninitialized pointer
*ptr = 10; // Dereferencing NULL pointer
// Correct
int *ptr = NULL;
ptr = (int *)malloc(sizeof(int));
*ptr = 10;
free(ptr);
|
|
|
Using = instead of == in C |
Posted by: Qomplainerz - 07-26-2023, 07:09 AM - Forum: C 18 Tutorials
- No Replies
|
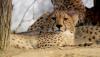 |
Assigning a value using = instead of comparing with == in conditional statements.
// Incorrect
int x = 5;
if (x = 10) {
printf("x is 10\n");
}
// Correct
int x = 5;
if (x == 10) {
printf("x is 10\n");
}
|
|
|
Incorrect loop conditions in C |
Posted by: Qomplainerz - 07-26-2023, 07:08 AM - Forum: C 18 Tutorials
- No Replies
|
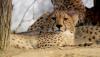 |
Using incorrect loop conditions can lead to infinite loops or incorrect program behavior.
// Incorrect
int i = 0;
while (i <= 5) {
printf("%d ", i);
// Missing increment of 'i' (i++ or i--)
}
// Correct
int i = 0;
while (i <= 5) {
printf("%d ", i);
i++;
}
|
|
|
Buffer overflow in C |
Posted by: Qomplainerz - 07-26-2023, 07:08 AM - Forum: C 18 Tutorials
- No Replies
|
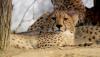 |
Writing more data into an array than it can hold can cause memory corruption and security vulnerabilities.
// Incorrect
char name[5];
strcpy(name, "John Doe"); // Buffer overflow
// Correct
char name[50];
strcpy(name, "John Doe");
|
|
|
Not initializing variables in C |
Posted by: Qomplainerz - 07-26-2023, 07:07 AM - Forum: C 18 Tutorials
- No Replies
|
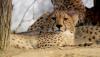 |
Using variables without initializing them can lead to unpredictable behavior.
// Incorrect
int x;
printf("%d\n", x); // The value of 'x' is undefined
// Correct
int x = 0;
printf("%d\n", x); // Output: 0
|
|
|
Missing Semi-colons in C |
Posted by: Qomplainerz - 07-26-2023, 07:06 AM - Forum: C 18 Tutorials
- No Replies
|
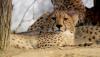 |
Forgetting to add a semicolon at the end of a statement can lead to compilation errors.
// Incorrect
int x = 10
int y = 20
// Correct
int x = 10;
int y = 20;
|
|
|
|