Welcome, Guest |
You have to register before you can post on our site.
|
Online Users |
There are currently 700 online users. » 0 Member(s) | 698 Guest(s) Bing, Yandex
|
Latest Threads |
SELECT statement with MS ...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:35 PM
» Replies: 0
» Views: 1,173
|
SELECT statement with the...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:31 PM
» Replies: 0
» Views: 614
|
Creating hyperlinks in HT...
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 01:23 PM
» Replies: 0
» Views: 931
|
What's new in HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:48 PM
» Replies: 0
» Views: 647
|
What is HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:43 PM
» Replies: 0
» Views: 593
|
Neck isometric exercises
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:44 AM
» Replies: 0
» Views: 890
|
Shoulder shrug
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 541
|
Neck retraction
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 500
|
Neck flexion and extensio...
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 588
|
Neck rotation
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 556
|
|
|
Typedef in C |
Posted by: Qomplainerz - 07-25-2023, 01:26 PM - Forum: C 18 Tutorials
- No Replies
|
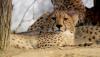 |
typedef is used to create aliases for data types.
typedef unsigned int uint;
int main() {
uint x = 10;
printf("%u\n", x); // Output: 10
return 0;
}
|
|
|
Enumerations in C |
Posted by: Qomplainerz - 07-25-2023, 01:25 PM - Forum: C 18 Tutorials
- No Replies
|
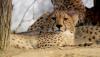 |
Enumerations define a set of named constants.
enum Color {
RED,
GREEN,
BLUE
};
int main() {
enum Color color = GREEN;
if (color == GREEN) {
printf("Color is Green\n");
}
return 0;
}
|
|
|
Preprocessor directives in C |
Posted by: Qomplainerz - 07-25-2023, 01:25 PM - Forum: C 18 Tutorials
- No Replies
|
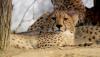 |
Preprocessor directives are instructions to the compiler before actual compilation.
// #define directive for a macro
#define PI 3.14159
// #include directive to include a header file
#include <stdio.h>
// Other preprocessor directives
#ifdef DEBUG
printf("Debug mode\n");
#else
printf("Release mode\n");
#endif
|
|
|
File pointers and streams in C |
Posted by: Qomplainerz - 07-25-2023, 01:24 PM - Forum: C 18 Tutorials
- No Replies
|
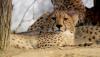 |
File pointers are used to interact with files.
FILE *file = fopen("data.txt", "r");
if (file != NULL) {
int ch;
while ((ch = fgetc(file)) != EOF) {
putchar(ch); // Output: Contents of the file
}
fclose(file);
}
|
|
|
Text and binary files in C |
Posted by: Qomplainerz - 07-25-2023, 01:24 PM - Forum: C 18 Tutorials
- No Replies
|
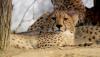 |
C supports both text and binary file handling.
// Writing to a binary file
int data[] = {10, 20, 30, 40, 50};
FILE *binary_file = fopen("data.bin", "wb");
if (binary_file != NULL) {
fwrite(data, sizeof(int), 5, binary_file);
fclose(binary_file);
}
// Reading from a binary file
int read_data[5];
binary_file = fopen("data.bin", "rb");
if (binary_file != NULL) {
fread(read_data, sizeof(int), 5, binary_file);
fclose(binary_file);
for (int i = 0; i < 5; i++) {
printf("%d ", read_data[i]); // Output: 10 20 30 40 50
}
}
printf("\n");
|
|
|
Reading and writing files in C |
Posted by: Qomplainerz - 07-25-2023, 01:23 PM - Forum: C 18 Tutorials
- No Replies
|
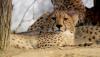 |
C provides functions for reading from and writing to files.
// Writing to a file
FILE *file = fopen("data.txt", "w");
if (file != NULL) {
fprintf(file, "Hello, World!");
fclose(file);
}
// Reading from a file
char buffer[100];
file = fopen("data.txt", "r");
if (file != NULL) {
fgets(buffer, 100, file);
printf("%s\n", buffer); // Output: Hello, World!
fclose(file);
}
|
|
|
Unions and their purposes in C |
Posted by: Qomplainerz - 07-25-2023, 01:22 PM - Forum: C 18 Tutorials
- No Replies
|
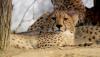 |
Unions are similar to structures, but they use the same memory location for all their members. Only one member can be active at a time.
union Data {
int i;
float f;
char str[20];
};
int main() {
union Data data;
data.i = 10;
printf("i: %d\n", data.i); // Output: 10
data.f = 3.14;
printf("f: %.2f\n", data.f); // Output: 3.14
strcpy(data.str, "Hello");
printf("str: %s\n", data.str); // Output: Hello
printf("Size of union Data: %lu bytes\n", sizeof(data)); // Output: 20 bytes
return 0;
}
|
|
|
Nested structures in C |
Posted by: Qomplainerz - 07-25-2023, 01:10 PM - Forum: C 18 Tutorials
- No Replies
|
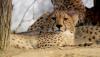 |
Structures can be nested within each other.
struct Address {
char city[50];
char state[50];
};
struct Person {
char name[50];
int age;
struct Address address;
};
int main() {
struct Person p1;
strcpy(p1.name, "John");
p1.age = 30;
strcpy(p1.address.city, "New York");
strcpy(p1.address.state, "NY");
printf("Name: %s, Age: %d, City: %s, State: %s\n", p1.name, p1.age, p1.address.city, p1.address.state);
return 0;
}
|
|
|
Defining and using structures in C |
Posted by: Qomplainerz - 07-25-2023, 11:43 AM - Forum: C 18 Tutorials
- No Replies
|
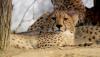 |
Structures are user-defined data types that allow you to group multiple variables of different data types under a single name.
struct Person {
char name[50];
int age;
float salary;
};
int main() {
struct Person p1;
strcpy(p1.name, "John");
p1.age = 30;
p1.salary = 50000.0;
printf("Name: %s, Age: %d, Salary: %.2f\n", p1.name, p1.age, p1.salary);
return 0;
}
|
|
|
Dynamic memory allocation in C (malloc, free) |
Posted by: Qomplainerz - 07-25-2023, 11:42 AM - Forum: C 18 Tutorials
- No Replies
|
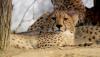 |
malloc() is used to allocate memory dynamically, and free() is used to release memory when it's no longer needed.
int *ptr = (int *)malloc(sizeof(int));
if (ptr != NULL) {
*ptr = 10;
printf("Value: %d\n", *ptr); // Output: 10
free(ptr); // Release memory
}
|
|
|
|