Welcome, Guest |
You have to register before you can post on our site.
|
Online Users |
There are currently 1480 online users. » 0 Member(s) | 1477 Guest(s) Yandex, Applebot, Bing
|
Latest Threads |
SELECT statement with MS ...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:35 PM
» Replies: 0
» Views: 1,465
|
SELECT statement with the...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:31 PM
» Replies: 0
» Views: 844
|
Creating hyperlinks in HT...
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 01:23 PM
» Replies: 0
» Views: 1,199
|
What's new in HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:48 PM
» Replies: 0
» Views: 893
|
What is HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:43 PM
» Replies: 0
» Views: 807
|
Neck isometric exercises
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:44 AM
» Replies: 0
» Views: 1,150
|
Shoulder shrug
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 735
|
Neck retraction
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 724
|
Neck flexion and extensio...
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 813
|
Neck rotation
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 787
|
|
|
C coding conventions and style guides |
Posted by: Qomplainerz - 07-25-2023, 01:32 PM - Forum: C 18 Tutorials
- No Replies
|
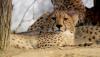 |
Following coding conventions improves code consistency within a project.
// C coding conventions example:
// 1. Use meaningful variable and function names
// 2. Indent code with 4 spaces
// 3. Add comments to explain complex logic
// 4. Declare variables at the beginning of the block
// 5. Use camelCase for variable and function names
// 6. Use UPPER_CASE for constants
// 7. Keep lines under 80 characters
|
|
|
Writing clean and maintainable code in C |
Posted by: Qomplainerz - 07-25-2023, 01:31 PM - Forum: C 18 Tutorials
- No Replies
|
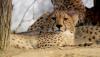 |
Writing clean and well-organized code enhances readability and maintainability.
// Bad coding style
int f(int x){int i=0;for(;i<x;++i)printf("%d ",i);return i;}
// Improved coding style
int print_numbers(int limit) {
for (int i = 0; i < limit; ++i) {
printf("%d ", i);
}
return limit;
}
|
|
|
Code optimization tips in C |
Posted by: Qomplainerz - 07-25-2023, 01:31 PM - Forum: C 18 Tutorials
- No Replies
|
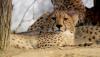 |
Code optimization aims to improve the performance and efficiency of the program.
#include <stdio.h>
int main() {
int x = 10;
if (x == 10) {
printf("x is 10\n");
}
return 0;
}
|
|
|
Debugging techniques and tools in C |
Posted by: Qomplainerz - 07-25-2023, 01:30 PM - Forum: C 18 Tutorials
- No Replies
|
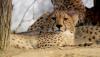 |
Debugging helps identify and fix errors in the code.
#include <stdio.h>
int main() {
int x = 10, y = 0;
int result = x / y; // Division by zero
printf("Result: %d\n", result); // Causes a runtime error (division by zero)
return 0;
}
|
|
|
Linking external libraries in C |
Posted by: Qomplainerz - 07-25-2023, 01:29 PM - Forum: C 18 Tutorials
- No Replies
|
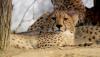 |
To use functions from external libraries, you need to link them during the compilation process.
// Compile with math library
// gcc main.c -lm -o main
#include <stdio.h>
#include <math.h>
int main() {
double result = pow(2, 3);
printf("2^3 = %.2f\n", result); // Output: 2^3 = 8.00
return 0;
}
|
|
|
Creating and using header files in C |
Posted by: Qomplainerz - 07-25-2023, 01:29 PM - Forum: C 18 Tutorials
- No Replies
|
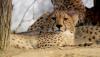 |
Header files contain function prototypes and other declarations.
// math_operations.h
#ifndef MATH_OPERATIONS_H
#define MATH_OPERATIONS_H
int add(int a, int b);
int subtract(int a, int b);
#endif
// math_operations.c
#include "math_operations.h"
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
// main.c
#include <stdio.h>
#include "math_operations.h"
int main() {
int result = add(10, 5);
printf("Addition: %d\n", result); // Output: 15
result = subtract(10, 5);
printf("Subtraction: %d\n", result); // Output: 5
return 0;
}
|
|
|
Overview of standard C library functions |
Posted by: Qomplainerz - 07-25-2023, 01:28 PM - Forum: C 18 Tutorials
- No Replies
|
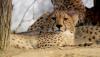 |
The C standard library provides many built-in functions to perform various operations.
#include <stdio.h>
#include <string.h>
int main() {
// String manipulation functions
char str1[] = "Hello";
char str2[10];
strcpy(str2, str1); // Copy str1 to str2
printf("%s\n", str2); // Output: Hello
// Mathematical functions
double result = sqrt(25);
printf("Square root of 25: %.2f\n", result); // Output: 5.00
return 0;
}
|
|
|
Handling errors and exceptions in C |
Posted by: Qomplainerz - 07-25-2023, 01:28 PM - Forum: C 18 Tutorials
- No Replies
|
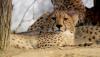 |
Error handling involves dealing with unexpected or exceptional situations.
#include <stdio.h>
#include <errno.h>
#include <string.h>
int main() {
FILE *file = fopen("non_existent_file.txt", "r");
if (file == NULL) {
printf("Error: %s\n", strerror(errno));
}
return 0;
}
|
|
|
Command-Line Arguments in C |
Posted by: Qomplainerz - 07-25-2023, 01:27 PM - Forum: C 18 Tutorials
- No Replies
|
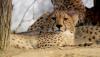 |
Command-line arguments allow passing arguments to a C program when executing it from the terminal.
int main(int argc, char *argv[]) {
if (argc > 1) {
printf("First argument: %s\n", argv[1]);
}
return 0;
}
|
|
|
Bit manipulation |
Posted by: Qomplainerz - 07-25-2023, 01:27 PM - Forum: C 18 Tutorials
- No Replies
|
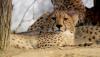 |
Bit manipulation involves performing operations at the bit level.
// Setting a bit using bitwise OR (|)
int num = 5; // Binary: 0101
int mask = 1; // Binary: 0001
int result = num | mask; // Binary: 0101 | 0001 = 0101
printf("%d\n", result); // Output: 5
|
|
|
|