Welcome, Guest |
You have to register before you can post on our site.
|
Online Users |
There are currently 1068 online users. » 0 Member(s) | 1065 Guest(s) Yandex, Bing, Applebot
|
Latest Threads |
SELECT statement with MS ...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:35 PM
» Replies: 0
» Views: 1,486
|
SELECT statement with the...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:31 PM
» Replies: 0
» Views: 861
|
Creating hyperlinks in HT...
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 01:23 PM
» Replies: 0
» Views: 1,227
|
What's new in HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:48 PM
» Replies: 0
» Views: 915
|
What is HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:43 PM
» Replies: 0
» Views: 825
|
Neck isometric exercises
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:44 AM
» Replies: 0
» Views: 1,178
|
Shoulder shrug
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 753
|
Neck retraction
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 735
|
Neck flexion and extensio...
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 836
|
Neck rotation
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 802
|
|
|
Declaring and defining functions in C |
Posted by: Qomplainerz - 07-25-2023, 11:01 AM - Forum: C 18 Tutorials
- No Replies
|
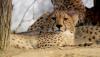 |
Functions are blocks of code that perform specific tasks. They need to be declared before using them.
// Function declaration
int add_numbers(int a, int b);
// Function definition
int add_numbers(int a, int b) {
return a + b;
}
|
|
|
Break and Continue statements in C |
Posted by: Qomplainerz - 07-25-2023, 11:00 AM - Forum: C 18 Tutorials
- No Replies
|
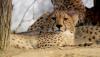 |
break is used to exit the loop prematurely, while continue is used to skip the current iteration and proceed to the next.
for (int i = 1; i <= 10; i++) {
if (i == 5) {
break; // Exit the loop when i is 5
}
printf("%d ", i);
}
// Output: 1 2 3 4
for (int i = 1; i <= 5; i++) {
if (i == 3) {
continue; // Skip the iteration when i is 3
}
printf("%d ", i);
}
// Output: 1 2 4 5
|
|
|
Looping structures in C (for, while, do-while) |
Posted by: Qomplainerz - 07-25-2023, 10:54 AM - Forum: C 18 Tutorials
- No Replies
|
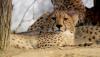 |
Loops allow you to execute a block of code repeatedly.
// Using a for loop to print numbers from 1 to 5
for (int i = 1; i <= 5; i++) {
printf("%d ", i);
}
printf("\n");
// Using a while loop to calculate the sum of numbers from 1 to 10
int sum = 0, i = 1;
while (i <= 10) {
sum += i;
i++;
}
printf("Sum: %d\n", sum);
// Using a do-while loop to print numbers from 1 to 5
int num = 1;
do {
printf("%d ", num);
num++;
} while (num <= 5);
printf("\n");
|
|
|
if-else statements in C |
Posted by: Qomplainerz - 07-25-2023, 10:52 AM - Forum: C 18 Tutorials
- No Replies
|
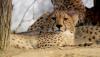 |
Conditional statements allow you to execute different code blocks based on certain conditions.
int num = 10;
if (num > 0) {
printf("Positive\n");
} else if (num < 0) {
printf("Negative\n");
} else {
printf("Zero\n");
}
|
|
|
Operators and Expressions in C |
Posted by: Qomplainerz - 07-25-2023, 10:51 AM - Forum: C 18 Tutorials
- No Replies
|
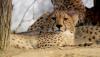 |
C supports various operators for performing arithmetic, comparison, logical, and bitwise operations.
int x = 10, y = 5;
int sum = x + y; // Arithmetic operator (+)
int is_equal = (x == y); // Comparison operator (==)
int is_true = (x && y); // Logical AND operator (&&)
|
|
|
Constants and Literals in C |
Posted by: Qomplainerz - 07-25-2023, 10:50 AM - Forum: C 18 Tutorials
- No Replies
|
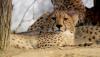 |
Constants are fixed values that cannot be modified during the program's execution. In C, you can use literals to represent constants.
#define PI 3.14159 // Declaring a symbolic constant using a #define directive
const int MAX_SIZE = 100; // Declaring a constant variable using the const keyword
|
|
|
Basic C syntax |
Posted by: Qomplainerz - 07-25-2023, 10:50 AM - Forum: C 18 Tutorials
- No Replies
|
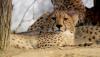 |
Data Types and Variables:
In C, you need to declare variables with their data types before using them. Common data types include int, float, char, double, etc.
int age; // Declaration of an integer variable
float pi = 3.14; // Declaration and initialization of a float variable
char letter = 'A'; // Declaration and initialization of a char variable
|
|
|
Writing your first "Hello, World!" program |
Posted by: Qomplainerz - 07-25-2023, 10:49 AM - Forum: C 18 Tutorials
- No Replies
|
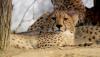 |
The "Hello, World!" program is a simple introductory program that prints the text "Hello, World!" to the console. Here's how you can do it:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
|
|
|
|