Welcome, Guest |
You have to register before you can post on our site.
|
Online Users |
There are currently 1400 online users. » 0 Member(s) | 1397 Guest(s) Yandex, Applebot, Bing
|
Latest Threads |
SELECT statement with MS ...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:35 PM
» Replies: 0
» Views: 1,465
|
SELECT statement with the...
Forum: MS Access SQL Tutorials
Last Post: Qomplainerz
07-27-2023, 03:31 PM
» Replies: 0
» Views: 844
|
Creating hyperlinks in HT...
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 01:23 PM
» Replies: 0
» Views: 1,199
|
What's new in HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:48 PM
» Replies: 0
» Views: 893
|
What is HTML5?
Forum: HTML5 Tutorials
Last Post: Qomplainerz
07-27-2023, 12:43 PM
» Replies: 0
» Views: 808
|
Neck isometric exercises
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:44 AM
» Replies: 0
» Views: 1,150
|
Shoulder shrug
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 735
|
Neck retraction
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:43 AM
» Replies: 0
» Views: 724
|
Neck flexion and extensio...
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 813
|
Neck rotation
Forum: Exercises
Last Post: Qomplainerz
07-27-2023, 11:42 AM
» Replies: 0
» Views: 787
|
|
|
Passing pointers to functions in C |
Posted by: Qomplainerz - 07-25-2023, 11:42 AM - Forum: C 18 Tutorials
- No Replies
|
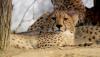 |
Pointers can be passed to functions to modify the original data.
void increment(int *num) {
(*num)++;
}
int main() {
int x = 5;
increment(&x);
printf("x: %d\n", x); // Output: 6
return 0;
}
|
|
|
Pointer arithmetic in C |
Posted by: Qomplainerz - 07-25-2023, 11:41 AM - Forum: C 18 Tutorials
- No Replies
|
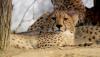 |
Pointers can be manipulated using arithmetic operations.
int numbers[] = {10, 20, 30, 40, 50};
int *ptr = numbers;
printf("%d\n", *ptr); // Output: 10
ptr++; // Move the pointer to the next element
printf("%d\n", *ptr); // Output: 20
|
|
|
Understanding pointers and memory addresses in C |
Posted by: Qomplainerz - 07-25-2023, 11:41 AM - Forum: C 18 Tutorials
- No Replies
|
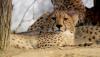 |
Pointers are variables that store memory addresses.
int num = 10;
int *ptr; // Declare a pointer
ptr = # // Assign the address of num to the pointer
printf("Value of num: %d\n", num); // Output: 10
printf("Address of num: %p\n", &num); // Output: Address in hexadecimal format
printf("Value of ptr: %p\n", ptr); // Output: Address of num
|
|
|
Working with strings in C |
Posted by: Qomplainerz - 07-25-2023, 11:40 AM - Forum: C 18 Tutorials
- No Replies
|
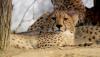 |
Strings are arrays of characters.
char name[] = "John";
printf("Name: %s\n", name); // Output: John
// String input using scanf()
char city[50];
printf("Enter your city: ");
scanf("%s", city);
printf("City: %s\n", city);
|
|
|
Multi-dimensional arrays in C |
Posted by: Qomplainerz - 07-25-2023, 11:40 AM - Forum: C 18 Tutorials
- No Replies
|
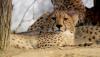 |
Multi-dimensional arrays are arrays of arrays.
int matrix[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
printf("%d\n", matrix[1][2]); // Output: 6
|
|
|
Declaring and initializing arrays |
Posted by: Qomplainerz - 07-25-2023, 11:39 AM - Forum: C 18 Tutorials
- No Replies
|
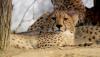 |
Arrays are collections of elements of the same data type.
// Declaring an array of integers
int numbers[5];
// Initializing an array with values
int primes[5] = {2, 3, 5, 7, 11};
|
|
|
Global and Local scope |
Posted by: Qomplainerz - 07-25-2023, 11:35 AM - Forum: C 18 Tutorials
- No Replies
|
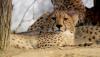 |
Variables declared outside any function have global scope and can be accessed throughout the program. Variables declared inside a function have local scope and can only be accessed within that function.
int global_var = 10; // Global variable
void my_function() {
int local_var = 5; // Local variable
printf("Global variable: %d\n", global_var);
printf("Local variable: %d\n", local_var);
}
int main() {
my_function();
return 0;
}
|
|
|
Function prototypes in C |
Posted by: Qomplainerz - 07-25-2023, 11:02 AM - Forum: C 18 Tutorials
- No Replies
|
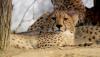 |
Function prototypes provide the compiler with information about the functions before their actual implementation.
// Function prototype
int add_numbers(int a, int b);
int main() {
int sum = add_numbers(10, 20);
printf("Sum: %d\n", sum);
return 0;
}
// Function definition
int add_numbers(int a, int b) {
return a + b;
}
|
|
|
|